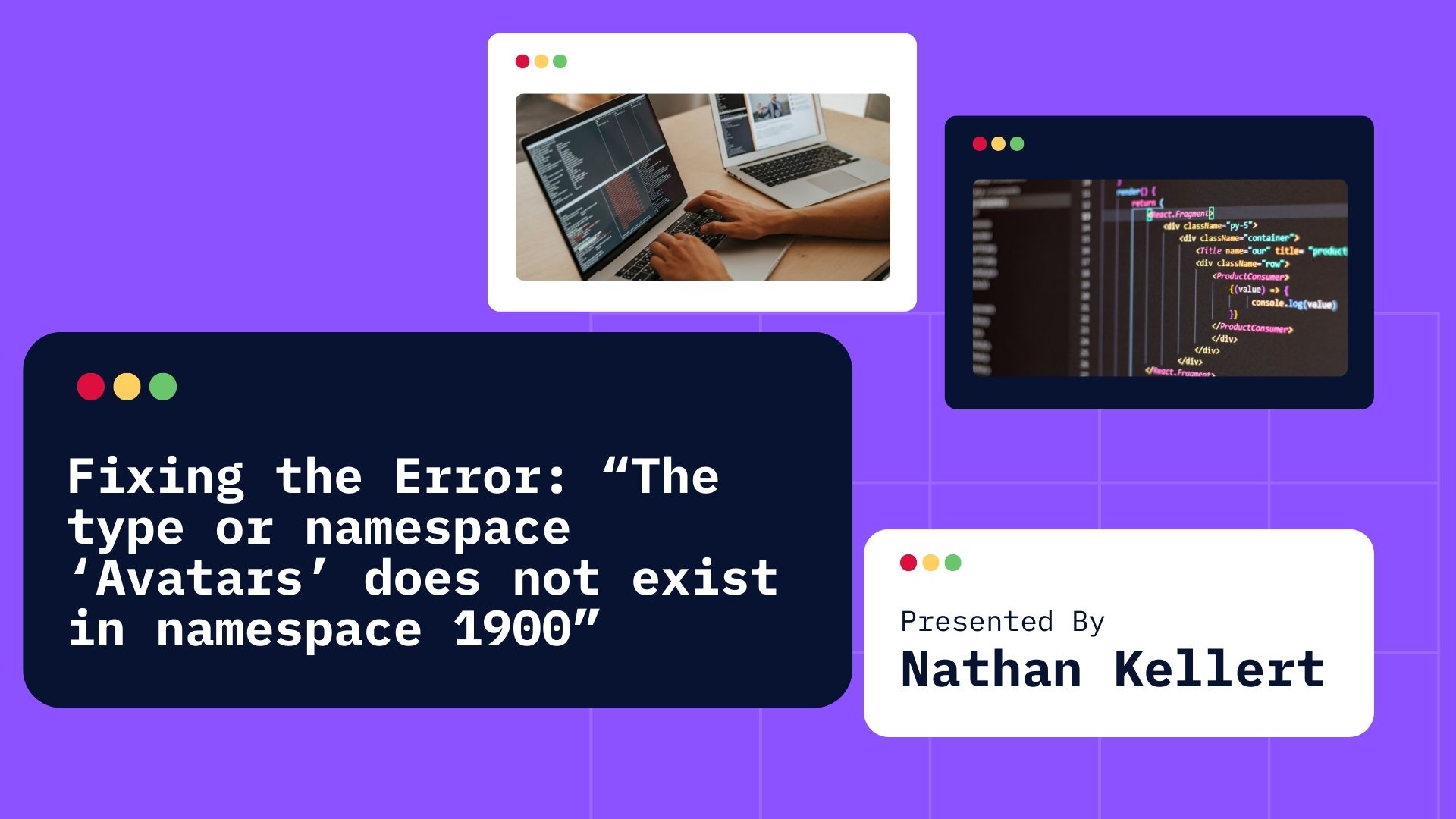
Are you encountering the error “The type or namespace ‘Avatars’ does not exist in namespace 1900” in your project? This issue usually occurs in C# (.NET) applications, especially when working with Unity, ASP.NET, or third-party libraries.
In this guide, we’ll explore:
✔️ What causes this error?
✔️ How to fix it step by step
✔️ Best practices to avoid similar issues
Let’s get started! 🚀
Table of Contents
1️⃣ What Causes This Error?
This error occurs when the compiler cannot find the ‘Avatars’ namespace in your project. Here are the most common reasons:
❌ Missing or incorrect using directive → The namespace isn’t imported at the top of your file.
❌ Missing or outdated library → The package containing ‘Avatars’ isn’t installed or is outdated.
❌ Incorrect assembly reference → Your project may not be referencing the required DLL correctly.
❌ Namespace mismatch → The namespace spelling or structure might be incorrect.
❌ Project build issues → Your project may need to be rebuilt or dependencies reloaded.
2️⃣ How to Fix “The type or namespace ‘Avatars’ does not exist in namespace 1900”
Here’s a step-by-step approach to fix this error in your c# file:
🔹 Step 1: Check Your Using Directive
Ensure you have the correct using
statement at the top of your C# file.
using Avatars;
If you’re using a specific library (like Unity’s Avatar system), you might need:
using UnityEngine;
using UnityEngine.Avatar;
💡 Pro Tip: If you’re unsure about the correct namespace, hover over Avatars
in your code and check for suggested fixes in Visual Studio or Rider.
🔹 Step 2: Verify That the Required Library Is Installed
If the error persists, the required library might be missing.
- In Unity, check if you have the right package installed via Package Manager.
- In ASP.NET / .NET projects, check your NuGet Packages and install the required package.
🔹 Install via NuGet (if applicable)
Try adding the required package using NuGet:
dotnet add package AvatarLibrary
Or manually install it in Visual Studio:
- Open Tools > NuGet Package Manager > Manage NuGet Packages for Solution.
- Search for the package related to Avatars.
- Click Install and rebuild your project.
🔹 Step 3: Check Your Assembly References
If your project depends on an external DLL, make sure it’s correctly referenced:
- Go to Solution Explorer in Visual Studio.
- Expand References and check if the required library is listed.
- If missing, right-click References → Add Reference → Select the missing DLL.
💡 Pro Tip: If you recently updated your project, an old reference might be broken. Try removing and re-adding the reference.
🔹 Step 4: Clean and Rebuild Your Project
Sometimes, your project might need a full rebuild to recognize dependencies properly.
- Close Visual Studio (or your IDE).
- Delete the
bin
andobj
folders from your project directory. - Open your IDE and rebuild the project.
In Visual Studio, you can do this via:
- Build > Clean Solution
- Build > Rebuild Solution
🔹 Step 5: Check for Namespace Typos
Make sure you’re using the correct namespace. Common mistakes include:
❌ using avatar;
(incorrect lowercase)
✔️ using Avatars;
(correct)
If the namespace is part of a third-party package, check the official documentation for the correct syntax.
3️⃣ Best Practices to Avoid This Error
✔️ Always check if the required package is installed before using a namespace.
✔️ Ensure proper references to external libraries (DLLs or NuGet packages).
✔️ Keep your packages up to date, but test compatibility before upgrading.
✔️ Rebuild your project after adding or modifying dependencies.
✔️ Use IntelliSense in Visual Studio to verify correct namespace imports.
Final Thoughts
If you’re facing “The type or namespace ‘Avatars’ does not exist in namespace 1900”, follow these steps:
✅ Check your using
directives for correctness.
✅ Verify that the required library or package is installed.
✅ Ensure correct assembly references if using external DLLs.
✅ Rebuild your project after making changes.
✅ Fix any namespace typos that might be causing the issue.
By following these steps, you should be able to resolve the issue and get your project running smoothly again! 🚀
If you’re troubleshooting C# or .NET issues, you might also find our guides on Fixing fatal: refusing to merge unrelated histories in Git and Handling ASP.NET Core App Startup Errors helpful
Still having trouble? Drop a comment with your specific error details, and I’ll help you out! 😊